NPTEL Intro to Programming Through C++ With the growth of Information and Communication Technology, there is a need to develop large and complex software. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the C++ programming language emerges as the best programming environment. This course provides an introduction to problem-solving and programming using the C++ programming language.
Intro to Programming Through C++ is a MOOC based course that is 12 weeks in duration and can fulfill the criteria of 4 credits in a year. You can visit the NPTEL SWAYAM platform and register yourself for the course. This course is brought to you by Prof. Partha Pratim Das received his BTech, MTech, and Ph.D. degrees in 1984, 1985, and 1988 respectively from IIT Kharagpur.
Intro to Programming Through C++ 2021 Details:-
Contents
- Who Can Join: First and second-year students in degree programs including Engineering and Science degree programs
- Requirements/Prerequisites: Standard XII in the Science stream
- INDUSTRY SUPPORT: Programming in C++ is so fundamental that all companies dealing with systems.
- These include – Microsoft, Samsung, Xerox, Yahoo, Google, IBM, TCS, Infosys, Amazon, Flipkart, etc.
An Intro To Programming Through C++ Assignment Week 12 Answers:-
Q1. We build a program that manages (very simplified) information about highways in the country. The program is to accept commands from the user and execute them until the user types a “T” (for terminate) command. Here are the different commands:
A <city1> <city2> <length>
This is for adding information to the data structure. The information to be added is: there is a highway from <city1> to <city2> of the given length.
HL <city1> <city2>
This should print the length of the highway from <city1> to <city2> if such a highway exists. If there is no such highway, -1 should be printed.
R <city1>
Code:-
double HL(string s,string d,vector<disttype> dTable)
{
for(auto& i: dTable){
if((i.origin==s && i.dest==d) || (i.origin==d && i.dest==s))
{
return i.distance;
}
}
return -1;
}
void R(string s,vector<disttype> dTable)
{
for(auto& i:dTable){
if(i.origin==s){
cout<<i.dest<<endl;
}
else if(i.dest==s){
cout<<i.origin<<endl;
}
}
}
Q2. You are to write a program that manages (very simplified) information about highways in thecountry. The information is stored in a map (explained later). The program is to acceptcommands from the user and execute them until the user types an “T” (for terminate) command.Here are the different commands:
● A <city1> <city2> length
This is for adding information to the map. The information to be added is: there is a highway from <city1> to <city2> of the given length
● HL <city1> <city2> This should print the length of the highway from <city1> to <city2>, if such a highway exists. If there is no such highway, -1 should be printed.
● R <city1> This should print the names of the cities that are reachable from <city1> using a single highway, each on a newline.
Code:-
int HL(string s,string d,map<string,map<string,int>>dTable)
{
if(dTable[s][d]!=0)
return dTable[s][d];
else if(dTable[d][s]!=0)
return dTable[d][s];
else
return -1;
}
void R(string s,map<string,map<string,int>>dTable){
for(auto i : dTable[s])
cout<<i.first<<endl;
}
An Intro To Programming Through C++ Assignment Week 11 Answers:-
Q1. C++ int variables usually use 4 bytes of space and it restricts their values to a range of [-2147483648,2147483647]. While this is a big range, in many applications, a much larger range of integers is desired. One can use long int and long long int for various purposes but we would like to design an unbounded integer class, BigInt.
Code:-
int BigInt::len () const
{
return digits.size();
}
BigInt::BigInt() {
}
BigInt::BigInt (string s) {
for(int i=0;i<s.size();i++) {
digits.push_back(s[i]-'0');
}
}
string BigInt::str() const {
string result="";
if(len()==0)
return "0";
else
{
for(int i=0;i<digits.size();++i)
{
result.append(to_string(digits[i]));
}
}
return result;
}
BigInt BigInt::operator + (const BigInt& a) const {
BigInt answer;
int i,carry=0;
if(digits.empty() && a.digits.empty())
return answer;
else if(digits.empty())
return a;
else if(a.digits.empty())
{
answer.digits=digits;
return answer;
}
long long int len1=digits.size(),len2=a.digits.size();
for(i=0;i<len1 && i<len2;i++)
{
int temp=digits[len1-(i+1)]+a.digits[len2-(i+1)]+carry;
if(temp>9)
{
answer.digits.push_back(temp-10);
carry=1;
}
else
{
answer.digits.push_back(temp);
carry=0;
}
}
if(i<len1)
{
for(;i<len1;i++)
{
if(digits[len1-(i+1)]+carry>9)
{
answer.digits.push_back(digits[len1-(i+1)]+carry-10);
carry=1;
}
else
{
answer.digits.push_back(digits[len1-(i+1)]+carry);
carry=0;
}
}
}
if(i<len2)
{
for(;i<len1;i++)
{
if(a.digits[len1-(i+1)]+carry>9)
{
answer.digits.push_back(a.digits[len1-(i+1)]+carry-10);
carry=1;
}
else
{
answer.digits.push_back(a.digits[len1-(i+1)]+carry);
carry=0;
}
}
}
if(carry==1)
answer.digits.push_back(1);
int s=answer.digits.size();
for(int i=0;i<s/2;i++)
{
int temp=answer.digits[i];
answer.digits[i]=answer.digits[s-(i+1)];
answer.digits[s-(i+1)]=temp;
}
return answer;
}
bool BigInt::operator < (const BigInt& a) const {
if(digits.size()<a.digits.size())
{
return true;
}
else if(digits.size()>a.digits.size())
{
return false;
}
int i=0;
while(digits[i]==a.digits[i])
{
i++;
}
if(i<digits.size() && digits[i]<a.digits[i])
return true;
return false;
}
Q2.You are provided with the details of various flights available on a day. This will befollowed by some queries. The queries will be of type: You are given the names of twocities, say city A and city B, and a departure time, say T. You have to write a program toprint the earliest time ON THE SAME DAY at which you can reach city B starting fromcity A using a single flight departing on or after time T. This is to be done for every query.
Code:-
#include<bits/stdc++.h>
using namespace std;
int main()
{
map<string, map<string, int> > departure;
map<string, map<string, int> > arrival;
string dc,ac;
int dt,at;
cin>>dc;
while(dc!="*")
{
cin>>dt>>ac>>at;
departure[dc][ac]=dt;
arrival[dc][ac]=at;
cin>>dc;
}
int n,t;
cin>>n;
while(n--)
{
cin>>dc>>ac>>t;
int th=0,tm=0,dh,dm,ah,am,i;
tm=t%100;
t=t/100;
th=t%100;
int d=departure[dc][ac];
dm=d%100;
d=d/100;
dh=d%100;
int a=arrival[dc][ac];
am=a%100;
a=a/100;
ah=a%100;
if((th<dh||(th==dh && tm<=dm))&&(ah>dh||(ah==dh && am>=dm)))
cout<<arrival[dc][ac]<<endl;
else
cout<<"-1"<<endl;
}
}
An Intro To Programming Through C++ Assignment Week 10 Answers:-
Q1. You are supposed to define a Histogram class to store Histogram objects. A histogram is a sequence of integers. The size of a histogram is the number of integers stored in it. Your class should have only and all of the following public methods :
- Histogram (int n) : A constructor which takes an integer n as argument and initializes a Histogram object with a sequence of n integers, indexed [0,n-1], all set to 0.
- Histogram () : A default constructor. This generates an empty histogram with an empty sequence. In essence, this is same as passing 0 to the previous constructor.
- void add (int i) : This member function adds 1 to the value stored at index i. If the index i is out of range, print a message saying “Invalid update performed” with a newline.
- void print() const : This member function prints the sequence in order 0 to n-1. Each integer is to be separated by a space and there should be a newline at the end. This is already implemented for you. If you are unfamiliar with the syntax, we recommend reading about it over the internet.
- Histogram operator + (const Histogram &h) const : An overloaded operator + to add 2 histograms. Addition of 2 histograms is defined as follows : suppose H1 has a size of n and H2 has a size m and suppose n>=m, then the first m entries of H3 = H1+H2 are the sum of entries of H1 and H2 and the remaining entries are copied from H1 as is.
Code:-
Q2. You are to write a program that manages a dictionary that stores English language words and for each word, what part of speech (POS) it is. As you might remember, noun, verb, adjective, … are POSs. For example, the dictionary might have the pairs (eat, verb), (pen, noun), (wise, adjective) in it. Initially, the dictionary is empty. You can add words into the dictionary or given a word, you can lookup its meaning. There are 4 commands: ADD, LOOKUP, ALL, END. Your program should read commands from the keyboard and perform the relevant action until the END command is received. Here are the descriptions of the commands
Code:-
An Intro To Programming Through C++ Assignment Week 09 Answers:-
Q1. Define a structure [1] for representing axis-parallel rectangles, i.e. rectangles whose sides are parallel to the axes. An axis parallel rectangle can be represented by the coordinates of the diagonally opposite points. Then, write a function [2] that takes an array of rectangles (axis parallel) and a point as arguments, and determine how many rectangles the point is inside of.
Code:-
struct Rectangle
{
int x1,y1,x2,y2;
};
int num_inside( Rectangle r[], int sz, int px, int py)
{
int count=0;
for(int i=0;i<sz;i++)
{
if(((r[i].x1==px && r[i].y1==py) && (r[i].x2==px || r[i].y2==py))||(r[i].x1<=px && r[i].y1<=py && r[i].x2>=px && r[i].y2>=py))
count++;
}
return count;
}
Q2. Design a class named Student. The class should have the following data members:
- char Name[100] ( This is to say that you will not deal with name longer than 99 characters)
- int EnglishMarks[4]
- int MathsMarks[4]
Code:-
class Student{
public:
char name[100];
int EnglishMarks[4];
int MathsMarks[4];
Student(){}
Student(char* nam_inp, int em[4], int mm[4])
{
int i;
for(i=0;nam_inp[i]!='\n';i++)
{
name[i]=nam_inp[i];
}
for(i=0;i<4;i++)
{
EnglishMarks[i]=em[i];
MathsMarks[i]=mm[i];
}
}
double avg(char subject)
{
double a;
if(subject=='E')
a=(double)(EnglishMarks[0]+EnglishMarks[1]+EnglishMarks[2]+EnglishMarks[3])/(double)4;
else if(subject=='M')
a=(double)(MathsMarks[0]+MathsMarks[1]+MathsMarks[2]+MathsMarks[3])/(double)4;
else
a=-1;
return a;
}
bool operator<(Student s2)
{
double sum1=EnglishMarks[0]+EnglishMarks[1]+EnglishMarks[2]+EnglishMarks[3]+MathsMarks[0]+MathsMarks[1]+MathsMarks[2]+MathsMarks[3];
double sum2=s2.EnglishMarks[0]+s2.EnglishMarks[1]+s2.EnglishMarks[2]+s2.EnglishMarks[3]+s2.MathsMarks[0]+s2.MathsMarks[1]+s2.MathsMarks[2]+s2.MathsMarks[3];
if(sum1<sum2)
return true;
else
return false;
}
};
An Intro To Programming Through C++ Assignment Week 08 Answers:-
Q1. There is a boy named Ali. He is a very curious child. His friend Shreya who likes to play with him read him a set of words and asked to find the maximum size subset of anagrams of the given set words. He, like always, got very excited and now wants you to solve the question for him.
Code:-
bool are_anagrams(char s1[5], char s2[5])
{
int first[26] = {0}, second[26] = {0};
for(int i=0;i<5;i++)
{
first[s1[i]-'a']++;
}
for(int i=0;i<5;i++)
{
second[s2[i]-'a']++;
}
for(int c = 0; c < 26; c++)
{
if(first[c] != second[c])
return false;
}
return true;
}
int max_anagram_subset(char string_set[][5], int n)
{
int count=1, max=1;
for(int i=0;i<n;i++)
{
for(int j=i+1;j<n;j++)
{
bool b=are_anagrams(string_set[i], string_set[j]);
if(b)
count++;
}
if(max<count)
max=count;
count=1;
}
return max;
}
Q2. You must have played the game of Xs and Os. If you have not, here are the rules. The game is played on a 3×3 square grid by two players (1 and 2). Player 1 is assigned ‘X’ and player 2 is assigned ‘O’. The game begins with the grid being empty. From here on, the players take turns and draw their character on the board, player 1 going first. A player who is able to secure a row, a column or a diagonal filled with only their characters before the other player can do so wins the game.
Code:-
int player,p=1,mark,pos,v,row,col,r,c,oner=0,onec=0,oned=0,twoc=0,twor=0,twod=0;
int board[n][n];
result=0;
for(int i=0;i<n;i++)
{
for (int j=0;j<n;j++)
{
board[i][j]=i*n+j;
}
}
for(int i=0;i<m; i++)
{
v=0;
player=(p % 2) ? 1 : 2;
cin>>pos;
for(r=0; r<n;r++)
{
for(c=0;c<n;c++)
{
if(board[r][c]==pos)
{
v=1;
row=r;
col=c;
}
}
}
if(v==1)
{
mark = (player == 1) ? 88 : 79;
board[row][col]=mark;
}
p++;
}
for(r=0;r<n-1;r++) {
for (c=0;c<n-1;c++) {
if(board[r][c]==board[r+1][c] && board[r][c]==88)
onec++;
if(board[r][c]==board[r+1][c] && board[r][c]==79)
twoc++;
if(board[r][c]==board[r][c+1] && board[r][c]==88)
oner++;
if(board[r][c]==board[r][c+1] && board[r][c]==79)
twor++;
if(board[r][r]==board[r+1][r+1] && board[r][c]==88)
oned++;
if(board[r][r]==board[r+1][r+1] && board[r][c]==79)
twod++;
}
}
if(oner>=n-1 || onec>=n-1 || oned>=n-1)
result=1;
else if(twor>=n-1 || twoc>=n-1 || twod>=n-1)
{
result=2;
}
else
result=0;
An Intro To Programming Through C++ Assignment Week 07 Answers:-
Q1. The input for this program consists of a number n, a sequence of n integers ao, a …0,-1 and an index d. You are supposed to print the prefix sum till index
d. Note that prefix sum till index d can be defined as a, (d included). You j=0
may assume that the size of an array can be a value that is known only during execution. For example, it is OK to write “int n; cin >>n; int A[n];”
Code:-
int main()
{
int n, d, i, sum=0;
cin>>n; int A[n];
for(i=0; i<n; i++) {
cin>>A[i];
} cin>>d;
for(i=0;i<=d;i++) {
sum+=A[i];
} cout<<sum<<endl;
return 0;
}
Q2. Write a program to determine whether a given sequence of integers s, s- S1s a palindrome. A palindrome is a sequence where,
S == S Vie {0, 1,. n – n-o-1 1}
Code:-
int main()
{
int n,i;
cin>>n;
int s[n];
for(i=0;i<n;i++)
{
cin>>s[i];
}
for(i=0;i<n/2;i++)
{
if(s[i]!=s[n-i-1])
break;
}
if(i==n/2)
cout<<"Yes"<<endl;
else
cout<<"No"<<endl;
return 0;
}
Intro To Programming Through C++ Assignment Week 06 Answers:-
C++ Week 6: Q1 –Write a program to print the term of the sequence which is given by nth the following rule:
Tn = 2 * Tn-1 + 3 * Tn-2
T0 and T1 will be provided by the user as input, so your program must take the two values input from the user.
Code:-
int fun(int t0, int t1, int n)
{
if(n==0)
return t0;
else if(n==1)
return t1;
else
return 2*fun(t0, t1, n-1) + 3*fun(t0, t1, n-2);
}
int main()
{
int t0, t1,n;
cin>>t0>>t1;
cin>>n;
cout<<fun(t0, t1,n)<<endl;
return 0;
}
C++ Week 6: Q2 – Write an overloaded function modified_power as follows. If the function is provided with two arguments say a and b, it computes a*b. When provided with three arguments say a,b,c. It computes a*(bc).
The return value of the function is of type integer and arguments of the function are also of type integer.
Code:-
#include<math.h>
int modified_power(int p, int q)
{
return(p*q);
}
int modified_power(int r,int s, int t)
{
return(r*pow(s, t));
}
Intro To Programming Through C++ Assignment Week 05 Answers:-
C++ Week 5: Q1 – The “signature” of a function is the first line which gives the return type, the name of the function and the parameters with their types. As an example the signature of the gcd function is
int gcd(int m, int n)
Write a function with the following signature
bool is_power_of_two(int n)
It should return true if the n is a power of 2. Otherwise, it should return false. As an example, for n = 28, the function should return false while for n = 32, it should return true.
Code:-
bool is_power_of_two(int n)
{
while(n>1)
{
if(n%2==0)
n=n/2;
else
break;
}
if(n==1)
return true;
else
return false;
}
C++ Week 5: Q2 – The “signature” of a function is the first line which gives the return type, the name of the function and the parameters with their types. As an example the signature of the gcd function is Write a function with the following signature to calculate interest given the principle P, the rate of interest r, and a character argument x which if “s” means we want simple interest and if “c” we want compound interest
The function performs 3 things1. It checks if the arguments P, r, x are valid. P is valid if it is > 0.
Argument r is valid if it is >0 and <1. Argument x is valid if it is either ‘c’ or ‘s’.2. If any of P, r, x are not valid, the function returns false.3. If the arguments P, r, x are valid, then the function calculates the simple interest if x = ‘s’, and compound interest if x = ‘c’. The function sets the reference parameter ans to the calculated interest and returns true.
Code:-
bool isInterest(double P, double r, int y, char x, double &ans)
{
if(P>0 && (r>0 && r<1))
{
if(x=='c')
{
ans=P*pow((1+r),y)-P;
return true;
}
else if(x=='s')
{
ans=P*r*y;
return true;
}
else
return false;
}
else
return false;
}
Intro To Programming Through C++ Assignment Week 04 Answers:-
C++ Week 4: Q1. Write a program that takes as input two numbers n (integer) and x (double), and prints arctan(x) evaluated by taking n terms of the Taylor series (using x0 =0):arctan(x) = x – x 3 /3 + x 5 /5 – x 7 /7 …
Code:-
int main()
{
int n;
double x, sum, term, i;
cin>>n>>x;
sum=x; term=x;
for(i=1;i<=n-1; i++)
{
term=-(term*x*x);
sum=sum+ (term/(2*i+1));
}
print(sum);
cout<<endl;
}
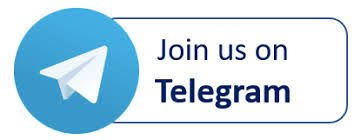
C++ Week 4: Q2. – Write a program to keep track of a match consisting of a series of games between two people: player A and player B, and report the outcome. The input consists of a sequence of letters A or B. If the input is A, it indicates that A has won a game. If it is B, then it indicates B has won a game. The first player to win 5 or more games with a difference of 2 or more games between him and his opponent wins the match. If no player wins the match in 20 games then the match is declared a tie after these 20 games have been played.
Code:-
int main()
{
int ac,bc,i;
char c;
ac=0;
bc=0;
for(i=1;i<=20; i++)
{
cin>>c;
if(c == 'A')
ac++;
else
bc++;
}
if( ac > bc)
cout<<"A"<<endl;
else if(ac < bc)
cout<<"B"<<endl;
else
cout<<"Tie"<<endl;
return 0;
}
C++ Assignment Week 03 Answers:-
C++ Week 3: Q1. Write a program to print the count of 1s in the binary representation of an integer N. Binary representation is the base-2 representation and only involves 0 and 1.
Answer/Code:-
int main()
{
int n,r,c=0;
cin>>n;
while(n>0)
{
r=n%2;
if(r==1)
c++;
n/=2;
}
cout<<c<<endl;
}
C++ Week 3: Q2. Write a program that is supposed to read n ( >= 3 ) numbers and print 1 if some 3 consecutive numbers are in arithmetic progression with some common difference (possibly 0), and print 0 otherwise.
Answer/Code:-
int main()
{
int n, d, n1, n2,c=0, p=-1;
cin>>n>>n2;
repeat(n-1)
{
cin>>n1;
d=n1-n2;
n2=n1;
if(d==p)
{
c++;
if (c==1)
{
cout<<"1\n";
exit(0);
}
}
else
p=d;
}
cout<<"0\n";
return 0;
}
C++ Assignment Week 02 Answers:-
C++ Week 2: Q1.Write a program to calculate the SPI(semester performance index) and CPI(Cumulative Performance Index) of a student taking N courses in a semester. Each course has a specified weight, termed as credit and a student secures a letter grade in that course as per the Grading System.
Answer/Code:-
int main()
{
int n, a[10],c=0,i,t;
char B[10];
double sp, s=0, cp, tt;
cin>>n;
for(i=0;i<n; i++)
cin>>B[i]>>a[i];
cin>>tt>>t;
for (i=0;i<n;i++)
{
c=c+a[i];
switch(B[i])
{
case 'A': s+=10*a[i]; break;
case 'B': s+=9*a[i]; break;
case 'C': s+=8*a[i]; break;
case 'D': s+=7*a[i]; break;
case 'E': s+=6*a[i]; break;
case 'F': s+=5*a[i]; break;
case 'G': s+=4*a[i]; break;
case 'H': s+=3*a[i]; break;
case 'I': s+=2*a[i]; break;
case 'J': s+=1*a[i]; break;
case 'K': s+=0*a[i]; break;
}
}
sp=s/c;
cp=(s+tt*t)/(c+t);
cout<<fixed<<setprecision(2)<<sp<<" "<<cp;
return 0;
}
C++ Week 2: Q2. Write a program which multiplies an N digit number M by a 1 digit number d, where N could be large, e.g. 1000. The input will be given as follows. First, the user gives d, then N and then the digits of M, starting from the least significant to the most significant.
The program must print out the digits of the product one at a time, from the least significant to the most significant.
Answer/code:-
int main()
{
int m,n,a[10],t[10],i,j,d,r=0;
cin>>m>>n;
for(i=0;i<n; i++)
cin>>a[i];
for(i=0;i<n;i++)
{
d=m*a[i]+r;
t[i]=d%10;
r=d/10;
}
if (r>0)
t[i++]=r%10;
for(j=0; j<i;j++)
cout<<t[j]<<"\n";
return 0;
}
Also check:-
- NPTEL » Introduction to Machine Learning Assignment 1 2021
- NPTEL » Soft Skill Development Assignment week 1 2021
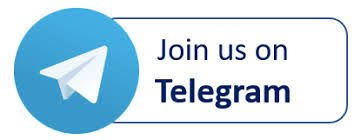
C++ Assignment Week 01 Answers:-
C++ Week 1: Q1. Write a program that reads in a number n and prints out asterisks(‘*’) in the shape of a rectangle having n rows and 2*n columns. Thus for n=3 your program should print
******
******
******
Answer/Code:-
using namespace std;
int main()
{
int n;
cin>>n;
for(int i=0;i<n;i++)
{
for(int j=0;j<2*n;j++)
{
cout<<"*";
}
cout<<endl;
}
return 0;
}
ALSO CHECK:-
C++ Week 1: Q2 – Write a program that reads in a number and prints out the letter L using ‘*’ characters with each line in the L having width n. Further, the length of the horizontal bar should be4n, and that of the vertical bar (i.e. not including the portion overlapping with the horizontal bar) should be 3n. Thus for n=3, your program should print
Answer/Code:-
using namespace std;
int main()
{
int n;
cin>>n;
for(int i=0;i<3*n;i++)
{
for(int j=0;j<n;j++)
cout<<"*";
cout<<endl;
}
for(int i=0; i<n;i++)
{
for(int j=0;j<4*n;j++)
cout<<"*";
cout<<endl;
}
return 0;
}
Find Other Quiz Here:
Amazon Fashion Quiz Answers & Win ₹1000
Amazon Great Indian Festival Quiz Answers: Win Rs. 50,000
NPTEL » Programming In Java Assignment week 6 Sep-2020
NPTEL » Programming In Java Assignment week 7 Sep-2020
NOTE: These codes are based on our knowledge. Answers might be incorrect, we suggest you to not the copy-paste answers blindly.
[foobar id=”1315″]